14 Feb 2017
I’ve been working on Adobe Animate CC quite a bit lately and wanted to create some custom Animate CC components. There hasn’t been too much documentation on this topic, so here’s what I gathered. I learnt a lot by looking at Adobe Animate CC’s default set of components which can be found in:
Windows
- Defaults: C:\Program Files\Adobe\Adobe Animate CC 2017\Common\Configuration\HTML5Components
- Custom: C:\Users\KahWee\AppData\Local\Adobe\Animate CC 2017\en_US\Configuration\HTML5Components
The rest of this is a guide to how Animate CC locates the contents in the directory and build up the Components panel you now see.
The slashes may get confusing, the convention of Adobe is to use the UNIX-style slashes /
instead of Windows \
as the directory separator so be sure to stick with it.
Diving into the main directories
Let’s look at the main dirctories in the defaults directory.
C:.
├───jqueryui
│ ├───assets
│ ├───jquery-ui-1.1
│ │ └───images
│ ├───locale
│ │ ├───...
│ │ └───zh_TW
│ └───src
├───lib
├───META-INF
├───sdk
├───ui
│ ├───assets
│ ├───locale
│ │ ├───...
│ │ └───zh_TW
│ └───src
└───video
├───assets
├───locale
│ ├───...
│ └───zh_TW
└───src
You see jqueryui
, lib
, META-INF
, sdk
, ui
and video
, of which jqueryui
, ui
and video
are shown in the Animate CC Components Panel here:
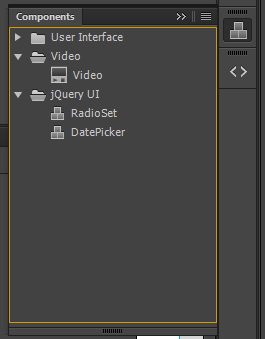
While I do not know why are Animate CC HTML5 Components ordered the way it is, a set of components qualifies as category set when it contains components.js
.
Learning from Video
If there’s a component to start learning from, I’ll humbly suggest “Video” as it is relatively trivial in terms of definition. Let’s start by dragging a “Video” from the Animate CC Components Panel.
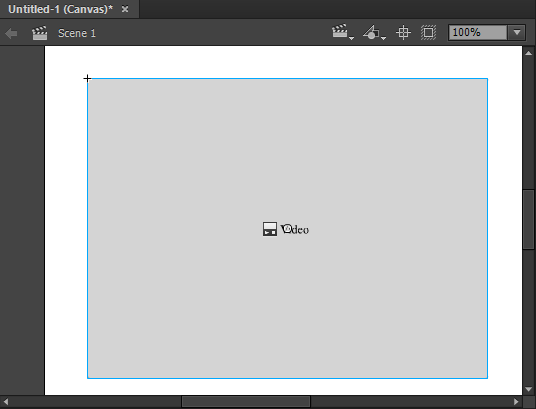
The component is available as it picks up contents from .\HTML5Components\video\components.js
which is a JSON file.
{
"category": "CATEGORY_VIDEO",
"components": [{
"className": "an.Video",
"displayName": "DISP_NAME_VIDEO",
"version": "1.0",
"source": "src/video.js",
"icon": "assets/SP_FLVPlayback_Sm",
"dimensions": [400, 300],
"dependencies": [
{"src": "../lib/jquery-2.2.4.min.js", "cdn": "https://code.jquery.com/jquery-2.2.4.min.js"},
{"src": "../sdk/anwidget.js"}
],
"properties": [
{"name": "PROP_SOURCE", "variable": "src", "type": "Video Content Path", "default": ""},
{"name": "PROP_AUTOPLAY", "variable": "autoplay", "type": "Boolean", "default": "true"},
{"name": "PROP_CONTROLS", "variable": "controls", "type": "Boolean", "default": "true"},
{"name": "PROP_MUTED", "variable": "muted", "type": "Boolean", "default": "false"},
{"name": "PROP_LOOP", "variable": "loop", "type": "Boolean", "default": "true"},
{"name": "PROP_POSTER", "variable": "poster", "type": "Image Path", "default": ""},
{"name": "PROP_PRElOAD", "variable": "preload", "type": "Boolean", "default": "true"},
{"name": "PROP_CLASS", "variable": "class", "type": "String", "default": "video"}
]
}]
}
In the above JSON file – oddly named components.json
, you see components
key holding an array. In the case of the “Video” category, there is only one component – “Video”. A good way to learn how to define the component is to modify and reload the Components Panel in ACC.
Defing properties in Video
There are 8 properties and they all correspond to what is in the Property Panel in order.
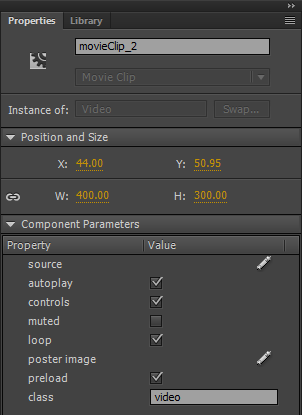
The properties[].name
placeholders can be defined in .\HTML5Components\video\locale\strings.json
. Here’s the contents for the file:
{
"locale": "en_US",
"language": "en",
"CATEGORY_VIDEO": "Video",
"DISP_NAME_VIDEO": "Video",
"PROP_SOURCE": "source",
"PROP_AUTOPLAY": "autoplay",
"PROP_CONTROLS": "controls",
"PROP_MUTED": "muted",
"PROP_LOOP": "loop",
"PROP_POSTER": "poster image",
"PROP_PRElOAD": "preload",
"PROP_CLASS": "class"
}
strings.json
lets you assign language set to the placeholders.
Types of properties
Video Content Path
: Lets you select a video file.
Boolean
: Reveal a checkbox to check and uncheck. The default
includes "true"
and "false"
(note that they are strings instead of booleans).
Image Path
: Lets you select an image file. Once you chosen the image, it gets copied into your images
directory.
String
: Lets you set a text field.
Collection
(Not shown)
Example of a property – class
class
is pretty straightfoward – let’s start by looking at how to define this.
{
"name": "PROP_CLASS",
"variable": "class",
"type": "String",
"default": "video"
}
Keys:
name
: What we have here is to define that the name of this property is using the PROP_CLASS
placeholder, of which you can locate in .\HTML5Components\video\locale\strings.json
file.
variable
: This is how to access the variable later in your code. It’s going to look like this – this._options['class']
, we will focus on this in a future post.
type
: This determines a text field to be used in the “Property Panel”
default
: This sets the default value of the text field to “video”
In this case, class
is used in defining the class for the “Video” component which can later be used for styling and more.
End
This post gets a little longer than I hope for. I’ll end here and guide you through the source code of the “Video” component in the next post. We’ll dive into components[].source
, located in .\HTML5Components\video\src\video.js
.
04 Sep 2016
Amazon Web Services has a product called Lambda that I had the opportunity to test out in a Hackathon. It made me rethink what micro-services is about.
The idea isn’t a new one, you send your source code up to AWS and it prepares it onto a server and exposes an index.handler
. What this handler does is that it exposes a set of API that you can call using REST.
The only downside is that you have to upload a ZIP source code all the time to deploy this. Or you could do this through pushing it to S3. In either cases you have this rudimentary interface to open up some really progressive ideas on micro-services.
I may be overthinking here. The terrible interface to update my files worked in its advantage, it encourages me to not deploy too many times. What I find myself doing is splitting up my code into small pieces, after all, each time some piece of code reaches a level of stability, it doesn’t have to be touched anymore. And that in turn reinforces the idea of micro-services – doing just one small bit super reliably.
Great job Amazon! I’m excited over what more it can do.
15 Aug 2016
So Microsoft added Ubuntu into Windows which is quite nice (besides the rather mouthful name). Let’s dig a little beneath the surface of things.
What it is not?
- It is not a Desktop. Duh. It says “Bash” after all. It didn’t occur to me until I installed it.
- It doesn’t integrate with Git for Windows or SSH agent in Windows
- It doesn’t mean you can open Linux programs outside of Bash on Ubuntu on Windows.
Where is it located in Windows?
Here: C:\Users\<YourUserName>\AppData\Local\lxss
For example, you usually /etc
directory is mapped here: C:\Users\KahWee\AppData\Local\lxss\rootfs\etc
.
I tried to manually edit the files here in Windows and it glitched out. Somehow the file disappeared from Bash on Ubuntu.
How do I get to my Windows drives?
It’s mounted in /mnt
. In the case for my F:
drive, it’s mounted here /mnt/f
. You have access to all your drives.
How do I restart Bash on Ubuntu?
You can restart only when you restart your Windows.
Can I open files with Windows applications?
Yes but it doesn’t really sync properly. I wanted a setup where I use Bash on Ubuntu for command line and the Windows file system to do web development. I couldn’t quite get used to this workflow.
Does Bash on Ubuntu support tabs?
Nope, much to my disappointment. I tend to open a lot of terminal tabs and I really miss that. Managing 10 of these terminal sessions with ALT+Tab
isn’t ideal.
Can I install zsh?
Yes! I did that. I’m not sure if it would glitch out.
Verdict
I like the idea but the moments when I lost files scare me a little. Until my confidence regains I will not be using this with serious work too soon. I’ll definitely be trying this again.
11 Aug 2016
I’m assuming you like to install PHP 5.6 with MySQL and want something more than MAMP. Good news is that Mac OS X El Capitan comes with Apache so you simply have to configure it.
To begin, you will need Homebrew, if you don’t already have that, install it here.
Installing MySQL and PHP 5.6
brew install mysql
brew install php56
brew install php56-mcrypt
Updating Apache httpd configuration
Next you will need to edit your Apache configuration. Open /private/etc/apache2/httpd.conf
for that.
sudo nano /private/etc/apache2/httpd.conf
Search for rewrite_module
and php5_module
.
You have to uncomment them and change them to the following:
LoadModule rewrite_module libexec/apache2/mod_rewrite.so
LoadModule php5_module /usr/local/opt/php56/libexec/apache2/libphp5.so
Notice that php5_module is referencing the Homebrew version. Note that your php.ini file can be found in: /usr/local/etc/php/5.6/php.ini
Starting the servers
Start MySQL this way:
Start Apache this way (you will need sudo):
Using VirtualHost
If you’re using VirtualHost, you will need to uncomment the following line in /private/etc/apache2/httpd.conf
. Search and uncomment this:
# Virtual hosts
Include /private/etc/apache2/extra/httpd-vhosts.conf
Next is to take a look at your VirtualHost file here /private/etc/apache2/extra/httpd-vhosts.conf
:
sudo nano /private/etc/apache2/extra/httpd-vhosts.conf
This is a sample of my VirtualHost:
<VirtualHost cors.kahwee:80>
DocumentRoot "/Users/kahwee/projects/cors"
ServerName cors.kahwee
ErrorLog "/private/var/log/apache2/cors.kahwee-error_log"
CustomLog "/private/var/log/apache2/cors.kahwee-access_log" common
</VirtualHost>
<Directory /Users/kahwee/projects/>
Options Indexes FollowSymLinks
AllowOverride All
Require all granted
</Directory>
Things to note in the above is the directory permissions. It may be different in your use case so be sure to set them properly. My project is using CakePHP is has the DocumentRoot in /Users/kahwee/projects/cors`
10 Aug 2016
(Disclaimer: I work in the advertising industry which utilize Flash for advertising opportunities.)
Chrome made the announcement that coming December, Chrome 55 will disable Flash by default and you’ll be prompted to enable Flash. This changes the game for a lot of advertising companies and publishing house that still rely on Flash to serve video.
Here’s the blog post:
Flash and Chrome
Today, more than 90% of Flash on the web loads behind the scenes to support things like page analytics. This kind of Flash slows you down, and starting this September, Chrome 53 will begin to block it. HTML5 is much lighter and faster, and publishers are switching over to speed up page loading and save you more battery life. You’ll see an improvement in responsiveness and efficiency for many sites.
In December, Chrome 55 will make HTML5 the default experience, except for sites which only support Flash. For those, you’ll be prompted to enable Flash when you first visit the site. Aside from that, the only change you’ll notice is a safer and more power-efficient browsing experience.
Source: Chrome
Flash is primarily used for advertisements and games, hardly for analytics purposes as far as I know.
Why browser and device vendors hate Flash so much?
Battery consumption and ability to optimize
Flash exists in a time where it is the only consistent way to play videos across platforms and browsers. The downside to this is that this hinders browser innovation to make videos run smoother. As the custodian of such a critical video distribution format, Adobe has done little to optimize video playing under browsers. Part of this is due to the separation of concerns enacted by the browsers plugin sandbox system.
The Adobe lockin
Essentially using Adobe Flash meant that you’re locked in their ecosystem. There’s a fundamental difference in ideology here where web browsers prefer work that has gone through collaboration.
As video becomes more and more prominent, browsers are starting to catch up with video streaming technologies. One of the reasons Flash stuck for so long is Mozilla’s insistence of not introducing DRM tools for quite a while. Netflix has their way when they supported browsers that works well with DRM. Today, Mozilla reversed the anti-DRM stance. With tighter integration, browsers introduce features such as tab-based muting.
Privacy and security issues
Flash implements its own set of cookies that is managed independently from the browser. Browsers aren’t able to clear cookies in there in the user’s command. This caused unscrupulous ad tracking companies to take advantage of this loophole and track user behaviors here.
Often battery is cited as an issue, but the other main reason will be privacy.
Who loses as Flash disappears?
Advertisers are key users of Flash. Blocking Flash does remove a lot of advertisements for the end users. Browsers get happy users, web content publishers lost their ability to monetize.
The reason why advertisers couldn’t switch easily is that designers couldn’t figure out how to design advertisements in JavaScript productively. For example, a designer who is using Animate CC is still crippled by the lack of support for JavaScript export and scripting abilities within Animate CC.
The losers at this time are designers who lost their workflow for using Flash to design interactive advertisements. Some advertising companies are losing out too and are transiting inventory toward Flash. It’s not going to be easy.