05 Aug 2016
CORS is a little hard to understand. I’ll give you a quick overview of what it is about.
There are two main modes of CORS:
- basic CORS which is
withCredentials
set to false
- preflight CORS which is
withCredentials
set to true
Let’s look at the first case.
Basic CORS
Basic CORS just want your server response to match an allowable origin. It isn’t uncommon to see servers returning with
Access-Control-Allow-Origin: *
This means it matches any domain name.
If the server returns three of the same header, like this:
Access-Control-Allow-Origin: *
Access-Control-Allow-Origin: *
Access-Control-Allow-Origin: *
…browsers interpret it as:
Access-Control-Allow-Origin: *, *, *
…which is invalid.
You will get the error message – “XMLHttpRequest cannot load http://example.com. The ‘Access-Control-Allow-Origin’ header contains multiple values ‘*, *, *’, but only one is allowed. Origin ‘http://example.com’ is therefore not allowed access.”
Preflight CORS
This has withCredentials
set to true and it disallows Access-Control-Allow-Origin
to be set to an *
.
When the request sends a header with a specified Origin
, it has to return the same value back as Access-Control-Allow-Origin
.
For example, in the request you have header:
Origin: http://example.com
Expected server response is:
Access-Control-Allow-Origin: http://example.com
Returning an *
is not allowed and you will be returned with an error.
Possible Apache2 (httpd) configuration could be:
SetEnvIf Origin "(^|\s)((https?:\/\/)?[\w-]+(\.[\w-]+)*\.?(:\d+)?(\/*)?)" AccessControlAllowOrigin=$0
Header always set Access-Control-Allow-Origin %{AccessControlAllowOrigin}e env=AccessControlAllowOrigin
<If "-z reqenv('AccessControlAllowOrigin')">
Header set Access-Control-Allow-Origin "*"
</If>
Possible nginx configuration could be:
add_header "Access-Control-Allow-Origin" $http_origin;
03 Aug 2016
I recently upgraded to Ubuntu 16.04 only to learn that it’s PHP7 now. Yes, I’m outdated. I wake up to finding my WordPress not working.
So we need to install php 7 and make some modifications.
sudo apt install php-fpm php-mysql
sudo apt install php7.0-xml
Configuring PHP7
Edit your php config file
sudo nano /etc/php/7.0/fpm/php.ini
Change the cgi.fix_pathinfo
setting to 0, you need to uncomment and change it.
After doing so, you need to restart php7.0-fpm
sudo systemctl restart php7.0-fpm
Configuring nginx
sudo nano /etc/nginx/nginx.conf
Add the following within the http
block to point to your newly installed php7.0-fpm:
upstream php {
server unix:/run/php/php7.0-fpm.sock;
}
This is my global/wordpress.conf
designed to be placed inside the individual server
blocks:
# WordPress single blog rules.
# Designed to be included in any server {} block.
rewrite /wp-admin$ $scheme://$host$uri/ permanent;
# Directives to send expires headers and turn off 404 error logging.
location ~* ^.+\.(ogg|ogv|svg|svgz|eot|otf|woff|mp4|ttf|rss|atom|jpg|jpeg|gif|png|ico|zip|tgz|gz|rar|bz2|doc|xls|exe|ppt|tar|mid|midi|wav|bmp|rtf)$ {
access_log off; log_not_found off; expires max;
}
# Pass all .php files onto a php-fpm/php-fcgi server.
location ~ [^/]\.php(/|$) {
if (!-f $document_root$fastcgi_script_name) {
return 404;
}
include snippets/fastcgi-php.conf;
fastcgi_pass php;
}
02 Aug 2016
Microsoft is preparing to release Windows anniversary updates alongside with Edge 14. What’s great is how much more compliant Edge is. Microsoft did a great job in ensuring this in the recent years:
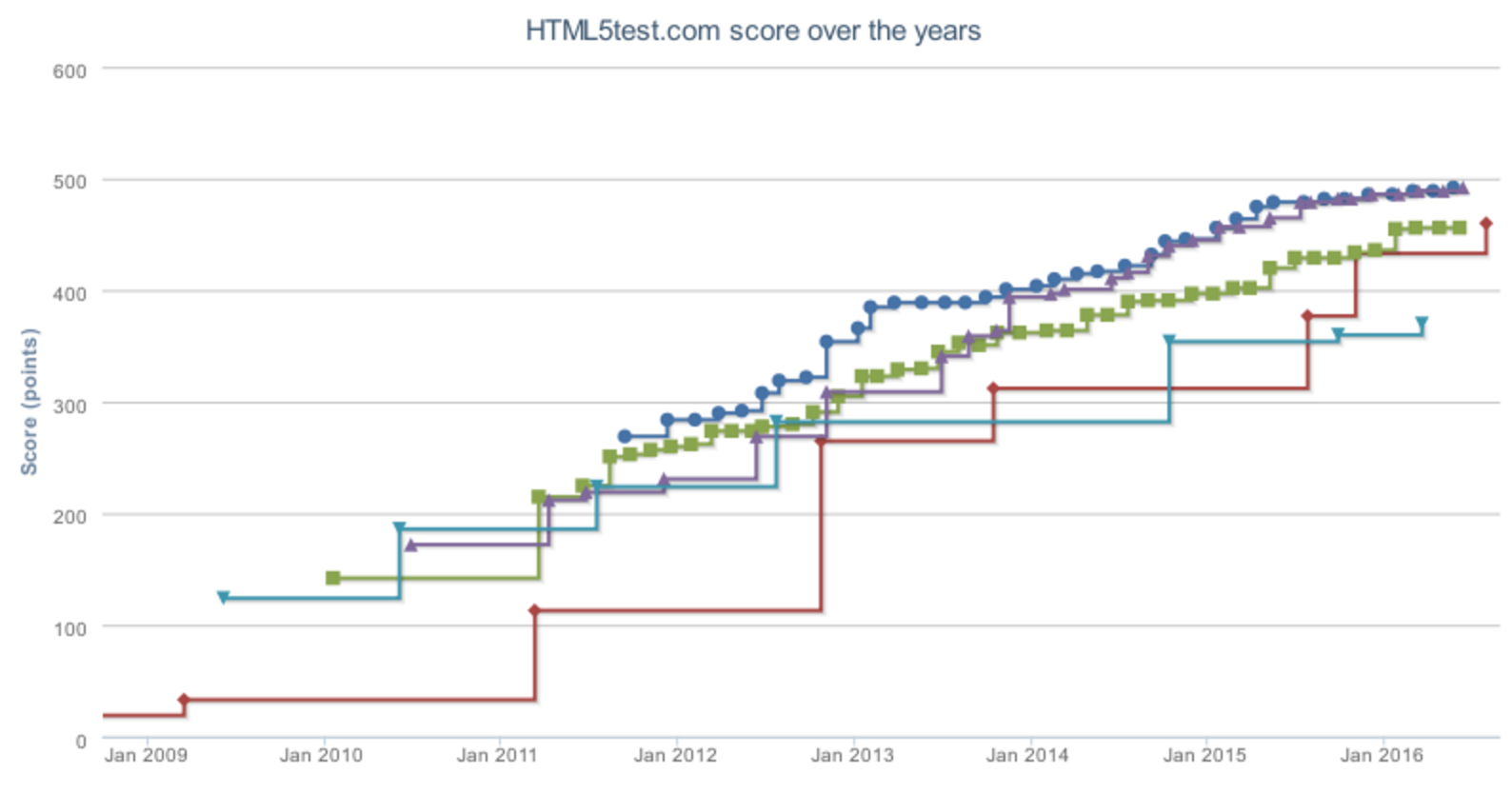
Edge14 (released tomorrow) is now right up there with Firefox on @html5test. 460 points. (473 with flags)
– David Storey
01 Aug 2016
I haven’t been maintaining my blogs well and they tend to get hacked due to my negligence. Each time there’s a hack I restart the blog and lose content that I spent ages constructing.
The losing content part always saddens me.
In order for better sadness management, I’ll shift my content to GitHub and write under Creative Commons.
Let’s hope this goes right this time around.
22 Jul 2016
This is excited – a major update of a longstanding testing tool.
Significant changes:
- Due to the increasing difficulty of applying security patches made within its dependency tree, as well as looming incompatibilities with Node.js v7.0, Mocha no longer supports Node.js v0.8.
.only()
is no longer “fuzzy”, can be used multiple times, and generally just works like you think it should.
- The
dot
reporter now uses more visually distinctive characters when indicating “pending” and “failed” tests.
See the changelog here
I’ve upgraded two repositories at work to Mocha v3. It is working right with the latest Karma and Chai.